需求分析
Icon组件的核心其实就是一个i标签,引入了一些字体图标,再添加一些样式,当然也可以自定义css样式。按照惯例,我们先来写一下Icon组件所需要的属性以及他们的类型有哪些。除此之外呢,我们还要找一些合适的字体图标,那么@fortawesome/fontawesome-svg-core
、@fortawesome/free-solid-svg-icons
和@fortawesome/vue-fontawesome
就很适合我们这个Icon组件。
代码实现
先来看看Icon组件可以接受哪些属性:
1 | import type { IconDefinition } from '@fortawesome/fontawesome-svg-core' |
组件结构
1 | <template> |
Icon组件的代码结构也很简单,它的核心就是一个<i>
标签,添加了一些属性和自定义的样式。
引入字体图标库
1 | import { library } from '@fortawesome/fontawesome-svg-core' |
在其他组件中使用Icon组件
在Button组件中使用
1 | <!-- Button.vue --> |
1 | <!-- 在App.vue中引入Button组件 --> |
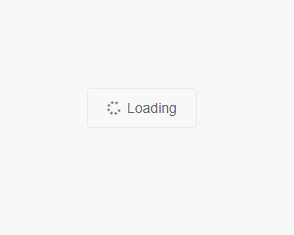